boost::asio::mockup_serial_port_service Class Reference
mockup_serial_port_service : A virtual serial port allowing to write cross platform unit tests of serial communicating application. More...
Inheritance diagram for boost::asio::mockup_serial_port_service:
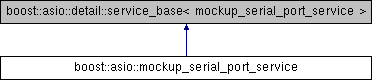
Public Types | |
typedef service_impl_type::implementation_type | implementation_type |
The type of a serial port implementation. | |
typedef service_impl_type::native_handle_type | native_type |
(Deprecated: Use native_handle_type.) The native handle type. | |
typedef service_impl_type::native_handle_type | native_handle_type |
The native handle type. | |
Public Member Functions | |
mockup_serial_port_service (boost::asio::io_service &io_service) | |
Construct a new serial port service for the specified io_service. | |
void | construct (implementation_type &impl) |
Construct a new serial port implementation. | |
void | move_construct (implementation_type &impl, implementation_type &other_impl) |
Move-construct a new serial port implementation. | |
void | destroy (implementation_type &impl) |
Destroy a serial port implementation. | |
boost::system::error_code | open (implementation_type &impl, const std::string &device, boost::system::error_code &ec) |
Open a serial port. | |
boost::system::error_code | assign (implementation_type &impl, const native_handle_type &handle, boost::system::error_code &ec) |
Assign an existing native handle to a serial port. | |
bool | is_open (const implementation_type &impl) const |
Determine whether the handle is open. | |
boost::system::error_code | close (implementation_type &impl, boost::system::error_code &ec) |
Close a serial port implementation. | |
native_type | native (implementation_type &impl) |
(Deprecated: Use native_handle().) Get the native handle implementation. | |
native_handle_type | native_handle (implementation_type &impl) |
Get the native handle implementation. | |
boost::system::error_code | cancel (implementation_type &impl, boost::system::error_code &ec) |
Cancel all asynchronous operations associated with the handle. | |
template<typename SettableSerialPortOption > | |
boost::system::error_code | set_option (implementation_type &impl, const SettableSerialPortOption &option, boost::system::error_code &ec) |
Set a serial port option. | |
template<typename GettableSerialPortOption > | |
boost::system::error_code | get_option (const implementation_type &impl, GettableSerialPortOption &option, boost::system::error_code &ec) const |
Get a serial port option. | |
boost::system::error_code | send_break (implementation_type &impl, boost::system::error_code &ec) |
Send a break sequence to the serial port. | |
template<typename ConstBufferSequence > | |
std::size_t | write_some (implementation_type &impl, const ConstBufferSequence &buffers, boost::system::error_code &ec) |
Write the given data to the stream. | |
template<typename ConstBufferSequence , typename WriteHandler > | |
BOOST_ASIO_INITFN_RESULT_TYPE (WriteHandler, void(boost::system::error_code, std::size_t)) async_write_some(implementation_type &impl | |
Start an asynchronous write. | |
const ConstBufferSequence | BOOST_ASIO_MOVE_ARG (WriteHandler) handler) |
template<typename MutableBufferSequence > | |
std::size_t | read_some (implementation_type &impl, const MutableBufferSequence &buffers, boost::system::error_code &ec) |
Read some data from the stream. | |
template<typename MutableBufferSequence , typename ReadHandler > | |
BOOST_ASIO_INITFN_RESULT_TYPE (ReadHandler, void(boost::system::error_code, std::size_t)) async_read_some(implementation_type &impl | |
Start an asynchronous read. | |
const MutableBufferSequence | BOOST_ASIO_MOVE_ARG (ReadHandler) handler) |
Public Attributes | |
const ConstBufferSequence & | buffers |
const MutableBufferSequence & | buffers |
Detailed Description
mockup_serial_port_service : A virtual serial port allowing to write cross platform unit tests of serial communicating application.
This class can be used in your unit tests to simulate a boost::asio::serial_port.
Example
using namespace boost::asio;
boost::thread simulate_writing_device([](){
io_service ios;
basic_serial_port<mockup_serial_port_service> port{ios, "my_fake_serial_port"};
const std::string message = "Hello";
for (;;) {
boost::asio::write(port, buffer(message.data(), message.size()));
boost::this_thread::sleep_for(boost::chrono::seconds(1));
}
});
boost::thread simulate_reading_device([](){
io_service ios;
basic_serial_port<mockup_serial_port_service> port{ios, "my_fake_serial_port"};
for (;;) {
char message[5];
boost::asio::read(port, buffer(message, 5));
std::cout << "received : " << std::string(message, 5) << std::endl;
}
});
The documentation for this class was generated from the following file:
- pre/boost/asio/mockup_serial_port_service.hpp